Web Studio is here! An enhanced experience to make it easier to create, preview, and collaborate on your website contentLearn More
Implementing a Custom 404 Page with Next.js
When visiting a page that does not exist, you will receive an HTTP 404 status code and be redirected to a 404 Page. Next.js comes with a default 404 page. However, we can create a custom 404 Page and have the content on that page managed from Agility.
Updating [...slug].js
First, you'll want to update the getStaticPaths
function inside of the [...slug].js
file to filter out and remove the 404 and/or 500 Page out of the paths to build as part of this slug.
In [...slug].js
:
// Next.js will statically pre-render all the paths from Agility CMS
export async function getStaticPaths({ locales, defaultLocale }) {
//get the paths configured in agility
let agilityPaths = await getAgilityPaths({
preview: false,
locales,
defaultLocale,
});
//remove the 404 and 500 page out of the paths to build as part of this slug
const filteredPaths = agilityPaths.filter(p => p !== "/404" && p !== "/500")
return {
paths: filteredPaths,
fallback: true,
};
}
In the getStaticProps
function, you'll want to update the following code from this:
if (!agilityProps) {
// We throw to make sure this fails at build time as this is never expected to happen
throw new Error(`Page not found`);
}
to this:
if (!agilityProps || agilityProps.notFound) {
// We throw to make sure this fails at build time as this is never expected to happen
return {
notFound: true,
};
}
Adding a 404.js Page
Next, you'll want to create a file-based route for your 404 Page. Inside the /pages
directory, create a file named 404.js
with the following code:
import { getStaticProps as getSlugStaticProps } from './[...slug]';
import Layout from "components/common/Layout";
export async function getStaticProps ({
preview,
locale,
defaultLocale,
locales,
}) {
//pass the 404 path into our getStaticProps function from the slug
const params = { slug: [ '404' ] }
return getSlugStaticProps({
preview,
params,
locale,
defaultLocale,
locales,
} )
}
const AgilityPage = (props) => {
return <Layout {...props} />;
};
export default AgilityPage
The code above can also be used to create a 500 Error page, just create a JavaScript file named 500.js
, and change the slug param to 500
.
Creating the 404 Page in Agility
In Agility, create a new Page in your Sitemap named 404.
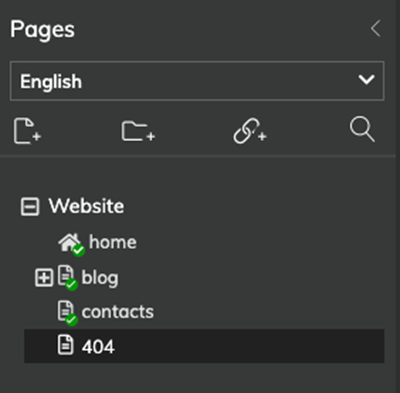
You can add what ever Page Modules you'd like, In this case, we will just be using a Rich Text Area.
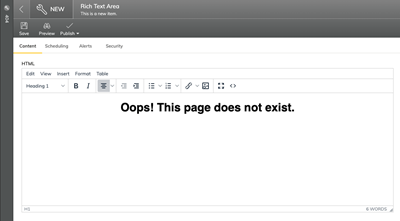
Once you've added your content, make sure your Page Module(s) and your 404 Page is Published.
You should now see your Custom 404 Page powered by Agility:
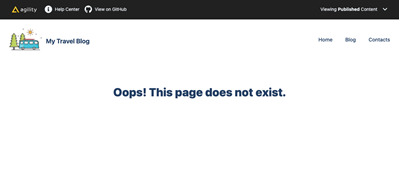