Visit Agility Academy to take courses and earn certifications. It's free, and you can learn at your own pace. Learn More
How Components Work in .NET
Components in Agility CMS are the functional components that make up a page. Editors use these to compose what type of content is on each page and in what order they appear.
Developers define what components are available in the CMS and what fields they have. In your .NET site, each component model defined in Agility CMS should correspond to 2 main components, your View and your ViewComponents.
Your ViewComponent, a
file, can be thought of as the "Backend" of the Component Model where most of the logic is happening. The View, a .cs
file, is the front-end component..cshtml
Generally speaking, if you don't have any modules defined or editors don't add modules to pages, then there won't be anything to output for your pages.
What is in a Component Model?
A Component Model in Agility CMS has a name, description, and fields.
The Name is a representation of the module's intended functionality, such as Posts Listing or Rich Text Area.
It is recommended that each module gets a description, this helps users understand when and where to use this definition.
Fields represent the content that can be managed by editors within the module. These fields are then used in code (passed as parameters) to display the content the editor intended.
An Example
in the agilitycms-dotnet-starter, the reference name of the Component Model is used to find a corresponding
and .cs
file that matches the same name. If a match is found, that component is dynamically imported and rendered..cshtml
For example, if a component has a reference name of RichTextArea in the CMS, then while the page is being rendered, it will look for ./Views/PageModules/RichTextArea.cshtml
and ./ViewComponents/PageModules/RichTextArea.cs
.
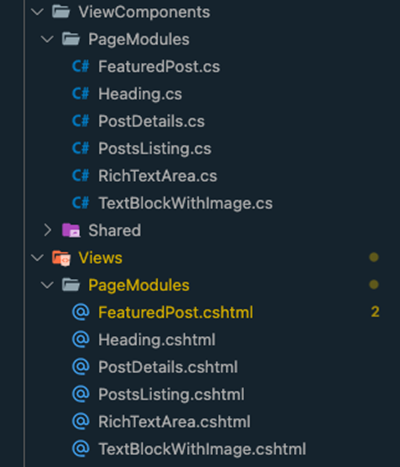
Internally, the Component Model will be dynamically imported and pass all the field values for that module as parameters, or in this case, @Models
.
RichTextArea.cshtml:
@model Agility.Models.RichTextArea
<div class="relative px-8">
<div class="max-w-2xl mx-auto my-12 md:mt-18 lg:mt-20">
<div class="prose max-w-full mx-auto">@Html.Raw(Model.TextBlob)</div>
</div>
</div>
RichTextArea.cs:
using System.Threading.Tasks;
using Agility.NET.FetchAPI.Helpers;
using Agility.NET.FetchAPI.Models;
using Microsoft.AspNetCore.Mvc;
namespace Agility.NET.Starter.ViewComponents.PageModules
{
public class RichTextArea : ViewComponent
{
public Task<IViewComponentResult> InvokeAsync(ModuleModel moduleModel)
{
var module = DynamicHelpers.DeserializeTo<Agility.Models.RichTextArea>(moduleModel.Module);
return Task.Run<IViewComponentResult>(() => View("/Views/PageModules/RichTextArea.cshtml", module));
}
}
}
How to Change the Fields
You can alter your fields at any time and the field values passed to your component will update automatically. If you change the structure of the component model then you need to generate your content models.
Generate your Content Models
cd
into the
directory.Agility.NET.AgilityCLI
- Overwrite the values in the
App.config
file (if not done already). - Run
.dotnet run update preview